Many people will learn just enough to be able to do their jobs, and then stop. Why should I bother learning new ways to do things, when I can get things done just fine right now?
This can be really apparent in computer programming. I once read about how most people find themselves a comfortable spot on the spectrum of computer programming language power. No matter where they are on that spectrum, they feel deep pity for people using less powerful tools, and they feel deep mystification about why anyone would need anything more powerful than the tools they have. (I can’t remember the reference. If someone knows what I’m talking about, tell me so I can cite it!)
Behold, the parable of the programmer. See if you can find yourself in this story.
A student is calculating a linear regression, fitting a straight line through a handful of data points. The textbook says that the best fit is the one that minimizes the sum of the squares of the deviations between the data points and the fit line. The student eyeballs a best fit, measures its slope, then computes the sum of squares with a pen and paper.
A second student taking the same class happens to see the first student in the library, undergoing this drudgery. “Why not use a calculator to make some of this easier?” they ask.
“Thanks, but I don’t need a calculator,” the first student says. “I’m just going to do this one regression; it will be more work than I need to figure out how to use that calculator.” The second student shrugs and starts their own homework, using a calculator.
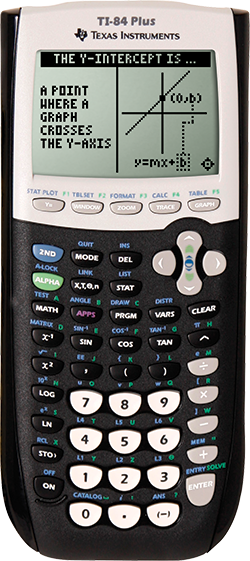
A third student wanders by, and sees the second student hunched over their calculator. “Hey, did you know you can write computer programs on those things? I wrote a program: given a slope, the sum of squares comes right out. So now I just need to punch in a few numbers, to figure out if my slope is the best fit. I can show you how!”
“No thanks,” says the second student. “It sounds like it will take me more time to learn how to write those programs, than to just use the tried-and-true: plus, minus, multiply, divide.” The third student shrugs and sits down to do their regression homework.
A fourth student sees the third. “Hey,” they say, “I was doing my homework like you’re doing it, but then someone showed me that you can write a program on the calculator, with this thing called a for-loop. Now I can just push one button, and get many sums of squares, for many slopes. By changing a single number in the program, I can check five slopes, ten slopes, or a thousand slopes! I can show you how?”
“No thanks,” says the third student. “I’m not really sure why I would need that. I mean, I’m only going to check five or ten slopes to find the best fit.” The fourth student shrugs, and walks off, to start their own homework.
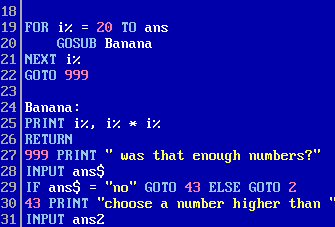
The fifth student asks the fourth, “Have you tried writing your regression in a macro or a subroutine? This way, if you ever want to change the covariates in your regression model, you only need to change one or two words in the input formula.” The fourth student says, “No thanks. I’m really only going to try to run one or two sets of covariates, so I’m not sure it’s worth my time to figure out how to do that.”
The sixth student asks the fifth, “How about writing your regression as a pure function, with an encapsulated namespace and no side effects?”
The fifth student says, “No thanks. I heard about pure functions in math class. Right now, my subroutine runs my commands to the computer, step-by-step. I’m not sure why reconceptualizing a ‘regression’ as a deterministic relationship between the input data and the output coefficients will help me at all. I mean, a program is something I ‘run,’ right? So why should I bother thinking about it as anything else, other than a series of operations run in sequence?”
The seventh student asks the sixth, “Have you thought about writing the regression model as a class? Then you can use object inheritance and common APIs, so you can swap in different linear models in your analytical pipeline, by just changing one or two words in your script. Like, you can go from linear regression to logistic regression by just changing that one word!”
The sixth student says, “No thanks. I opened a Java textbook once. It didn’t make any sense. First of all, the program doesn’t start at the top, it starts wherever ‘main’ is defined. And then that line has the mysterious incantation ‘public, static, void, main, string, args.’ Too crazy for me. Python looks a little easier, but I’m still just not sure I need objects. Like, in R, I can call lm() for linear regressions and glm() for logistic regressions. So why do I need something crazy like sklearn.linear_model.LinearRegression()?”
The eighth student asks the seventh, “I see you’re writing some code in Python and R. Have you ever thought about learning a strongly typed language? It’s not just about the performance improvement that comes from compilation; you also get added layers of debugging and annotation that make your programs really safe!”
The seventh student says, “No thanks. I opened a C++ textbook once. I’m pretty sure malloc is the name of the hammer that waled on my brain every second I had that book open.”
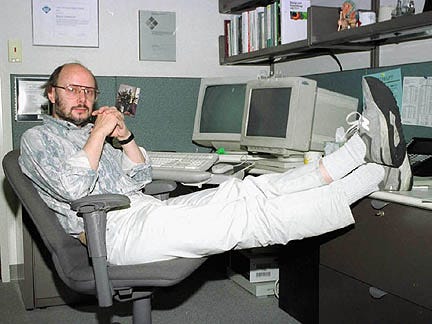
The ninth student asks the eighth, “Have you ever considered that a function of more than one variable, can really be thought of as a function of one variable, that returns a function, that takes the rest of those variables as inputs? After I learned this, I realized how I’m already implicitly using partial applications in my programs, but in inconsistent ways, which made it harder to reason about the types of my objects!”
The eighth says, “Please go away and stop telling me such things, or I’ll throw a monad at you.”
THE END
Did you relate to any of the students? My guess is that you found the students below you a little narrow-minded and the ones above you a bit ridiculous. The question is, if you move “up” one step on the ladder, would you still feel the same way?
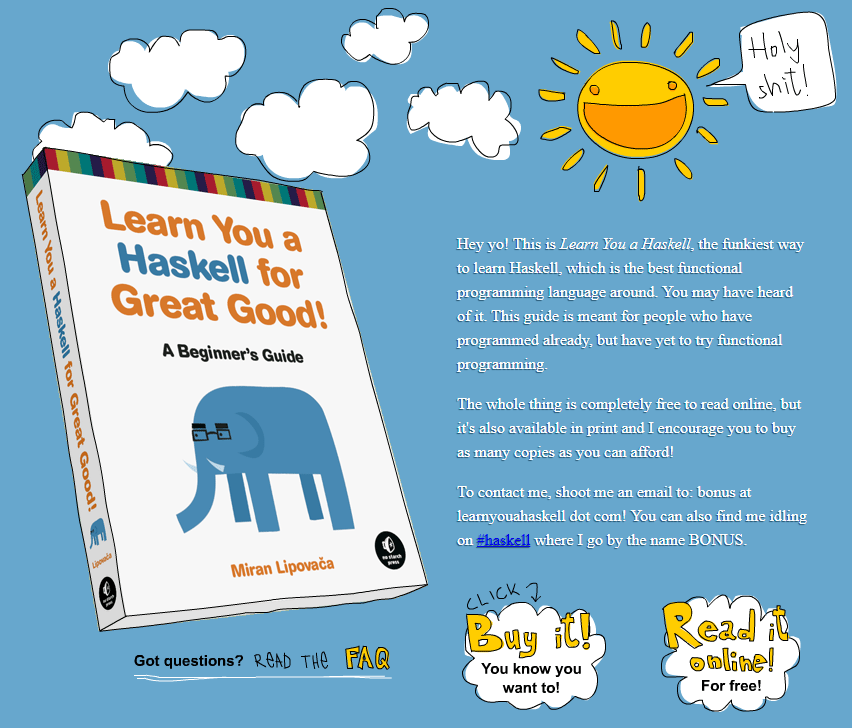